I forget exactly how I came across this puzzler. It has been solved by deductive reasoning, brute force, and in a variety of programming languages. I felt compelled to develop my own solution in Python. The problem is as follows (taken from the Car Talk website).
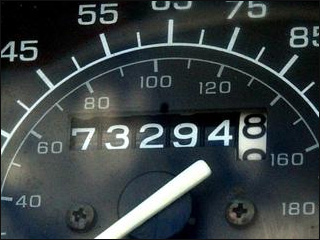
"I noticed that the last 4 digits were palindromic. I drove a mile, and the last 5 were palindromic. I drove another mile and the middle 4 were palindromic, and the ends were not involved. And then one mile later, all 6 digits were palindromic."
First, I wondered if leading zeroes on the odometer counted as eligible digits for comparison. As it would turn out, it makes no difference. My solution incorporated this regardless. Another interesting bit is that there are technically two solutions that satisfy the conditions outlined by the author, though the second solution is much less elegant and for the first number in the sequence the first five digits are palindromic. After refreshing my memory regarding splice syntax in Python, I was on my way. Running the following code produces two results: 198,888 and 199,999.
import math def ispalindrome(text): plen = math.floor(len(text) / 2) + 1 if text[:plen-1] == text[:-plen:-1]: return True else: return False for i in range(100000, 1000000): reading = str(i).zfill(6) if len(reading) == 6: condition_1 = str(i).zfill(6)[2:] condition_2 = str(i+1).zfill(6)[1:] condition_3 = str(i+2).zfill(6)[1:5] condition_4 = str(i+3).zfill(6) if ispalindrome(condition_1) \ and ispalindrome(condition_2) \ and ispalindrome(condition_3) \ and ispalindrome(condition_4): print("Odometer initially read: " + str(i))
For the more elegant answer of 198888, the sequence is 198888 -> 198889 -> 198890 -> 198891. The less elegant answer of 199999 yields a sequence of 199999 -> 200000 -> 200001 -> 200002. In all likelihood, the author was referencing the first solution of 198,888 or else he would have specified that the last five digits were palindromic to start the sequence. There is also a sense of "less palindromic" when dealing with numbers that are mostly identical to take into account.